In today’s digital landscape, web applications rely heavily on databases for data storage and management. Python, with its simplicity and versatility, has become a preferred choice for web developers. This article explores how to establish Python database connectivity for your website, covering essential libraries, best practices, and SEO optimization techniques to ensure your content is both informative and easily discoverable.
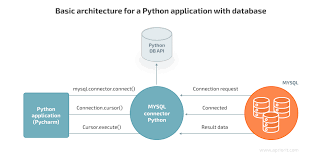
What is Database Connectivity?
Database connectivity refers to the ability of a programming language to communicate with a database management system (DBMS). For web applications, this means allowing users to perform operations such as creating, reading, updating, and deleting data (often referred to as CRUD operations).
Why Use Python for Database Connectivity?
Python’s popularity in web development stems from its powerful frameworks, ease of use, and extensive libraries. Some key advantages include:
- Simplicity: Python’s syntax is clear and intuitive, making it accessible for beginners.
- Versatile Frameworks: Frameworks like Django and Flask provide built-in support for database interactions.
- Rich Ecosystem: A plethora of libraries and tools simplify database connections.
Setting Up Python Database Connectivity
1. Choosing the Right Database
Before diving into coding, choose a database that suits your application’s needs. Popular options include:
- SQLite: Lightweight and serverless, ideal for small applications.
- PostgreSQL: A powerful open-source database with advanced features.
- MySQL: Widely used and well-supported, great for web applications.
2. Installing Required Libraries
To connect Python with your chosen database, you’ll need to install the relevant libraries. Here’s how to do it:
For SQLite:
bashCopy codepip install sqlite3
For PostgreSQL:
bashCopy codepip install psycopg2
For MySQL:
bashCopy codepip install mysql-connector-python
3. Connecting to the Database
Once the libraries are installed, you can establish a connection. Here’s a basic example for each database:
SQLite:
pythonCopy codeimport sqlite3
# Connect to SQLite database
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
PostgreSQL:
pythonCopy codeimport psycopg2
# Connect to PostgreSQL database
conn = psycopg2.connect(
dbname='yourdbname',
user='yourusername',
password='yourpassword',
host='localhost',
port='5432'
)
cursor = conn.cursor()
MySQL:
pythonCopy codeimport mysql.connector
# Connect to MySQL database
conn = mysql.connector.connect(
user='yourusername',
password='yourpassword',
host='localhost',
database='yourdbname'
)
cursor = conn.cursor()
4. Performing CRUD Operations
After establishing a connection, you can perform CRUD operations:
Create:
pythonCopy code# Create a table
cursor.execute('''CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT)''')
Read:
pythonCopy code# Read data
cursor.execute('SELECT * FROM users')
rows = cursor.fetchall()
for row in rows:
print(row)
Update:
pythonCopy code# Update data
cursor.execute('UPDATE users SET name = ? WHERE id = ?', ('Alice', 1))
conn.commit()
Delete:
pythonCopy code# Delete data
cursor.execute('DELETE FROM users WHERE id = ?', (1,))
conn.commit()
5. Closing the Connection
Always ensure to close the database connection to free up resources:
pythonCopy codecursor.close()
conn.close()
Best Practices for Database Connectivity
- Use Environment Variables: Store database credentials in environment variables to enhance security.
- Implement Error Handling: Use try-except blocks to manage exceptions effectively.
- Optimize Queries: Always analyze and optimize your SQL queries for performance.
Conclusion
Python database connectivity is a fundamental skill for web developers. By leveraging its powerful libraries and frameworks, you can create dynamic web applications that interact seamlessly with databases. Implement the best practices outlined above, and don’t forget to optimize your content for search engines to maximize visibility. Whether you’re building a small project or a large-scale application, Python is an excellent choice for effective database connectivity.
By following these guidelines, you can ensure your web application is robust, efficient, and ready for the demands of modern users.
For more check out our another platform on YouTube.
Blue Techker This is my first time pay a quick visit at here and i am really happy to read everthing at one place
hiI like your writing so much share we be in contact more approximately your article on AOL I need a specialist in this area to resolve my problem Maybe that is you Looking ahead to see you
Usually I do not read article on blogs however I would like to say that this writeup very compelled me to take a look at and do it Your writing style has been amazed me Thank you very nice article
Noodlemagazine Pretty! This has been a really wonderful post. Many thanks for providing these details.
Noodlemagazine naturally like your web site however you need to take a look at the spelling on several of your posts. A number of them are rife with spelling problems and I find it very bothersome to tell the truth on the other hand I will surely come again again.
Noodlemagazine I very delighted to find this internet site on bing, just what I was searching for as well saved to fav
Noodlemagazine Great information shared.. really enjoyed reading this post thank you author for sharing this post .. appreciated